可绑定属性
所有公共自动实现的虚拟属性都被视为可绑定的。
C#
public virtual string Test { get; set; }
VB.NET
Public Overridable Property Test() As String
要禁止为此类属性生成可绑定属性,请按如下方式使用 Bindable 属性。
C#
[Bindable(false)]public virtual string Test { get; set; }
VB.NET
<Bindable(False)>Public Overridable Property Test() As String
框架会忽略带有支持字段的属性,您可以使用 BindableProperty 特性显式标记此类属性,以便仍然能够将它们用于数据绑定。
C#
using DevExpress.Mvvm.DataAnnotations;//. . .string test;[BindableProperty]public virtual string Test{get { return test; }set { test = value; }}
VB.NET
Imports DevExpress.Mvvm.DataAnnotations'. . .Private testField As String<BindableProperty>Public Overridable Property Test() As StringGetReturn testFieldEnd GetSet(ByVal value As String)testField = valueEnd SetEnd Property
属性依赖
属性可以在应用程序运行时更改其值,要跟踪这些更改并对其做出响应,请声明属性依赖项。属性依赖是一种在其相关属性发生变化或即将发生变化时自动执行的方法。 要实现此操作,必须调用On<Related_Property_Name>Changing或On<Related_Property_Name>Changed方法。
C#
public virtual string Test { get; set; }protected void OnTestChanged() {//do something}
VB.NET
Public Overridable Property Test() As StringProtected Sub OnTestChanged()'do somethingEnd Sub
On…Changed 和 On..Changing 方法也可以有一个参数,在这种情况下,参数将分别接收旧的或新的属性值。
C#
public virtual string Test { get; set; }protected void OnTestChanging(string newValue) {//do something}protected void OnTestChanged(string oldValue) {//do something}
VB.NET
Public Overridable Property Test() As StringProtected Sub OnTestChanging(ByVal newValue As String)'do somethingEnd SubProtected Sub OnTestChanged(ByVal oldValue As String)'do somethingEnd Sub
BindableProperty 属性也允许您使用具有不同名称的方法。
C#
[BindableProperty(OnPropertyChangingMethodName = "BeforeChange", OnPropertyChangedMethodName = "AfterChange")]public virtual string Test { get; set; }protected void BeforeChange() {//. . .}protected void AfterChange() {//. . .}
VB.NET
<BindableProperty(OnPropertyChangingMethodName := "BeforeChange", OnPropertyChangedMethodName := "AfterChange")>Public Overridable Property Test() As StringProtected Sub BeforeChange()'. . .End SubProtected Sub AfterChange()'. . .End Sub
命令
在 POCO ViewModels 中声明的所有具有零个或一个参数的公共无效方法都被视为命令。
C#
public void DoSomething(object p) {MessageBox.Show(string.Format("The parameter passed to command is {0}.", p));}
VB.NET
Public Sub DoSomething(ByVal p As Object)MessageBox.Show(String.Format("The parameter passed to command is {0}.", p))End Sub
名称以 …Command 结尾的方法会引发异常,您可以通过使用 Command 属性标记这些方法并通过 Name 参数设置适当的名称来强制框架将这些方法视为有效命令。
C#
using DevExpress.Mvvm.DataAnnotations;[Command(Name="DoSomething")]public void DoSomethingCommand(object p) {//do something}
VB.NET
Imports DevExpress.Mvvm.DataAnnotations<Command(Name := "DoSomething")>Public Sub DoSomethingCommand(ByVal p As Object)'do somethingEnd Sub
对于每个命令方法,框架都会生成相应的支持属性。 默认情况下,此属性以相关方法加上“Command”后缀命名。 您可以使用 Command 属性的 Name 参数为这个自动生成的支持属性保留另一个名称。
C#
[Command(Name = "MyMvvmCommand")]public void DoSomething(object p) {//do something}
VB.NET
'this command will be executed only if "p" equals 4Public Sub DoSomething(ByVal p As Integer)MessageBox.Show(String.Format("The parameter passed to command is {0}.", p))End SubPublic Function CanDoSomething(ByVal p As Integer) As BooleanReturn (2 + 2) = pEnd Function
具有其他名称的 CanExecute 方法仍然可以通过使用 Command 属性的 CanExecuteMethodName 参数绑定到命令。
C#
[Command(CanExecuteMethodName = "DoSomethingCriteria")]public void DoSomething(int p) {MessageBox.Show(string.Format("The parameter passed to command is {0}.", p));}public bool DoSomethingCriteria(int p) {return (2 + 2) == p;}
VB.NET
<Command(CanExecuteMethodName := "DoSomethingCriteria")>Public Sub DoSomething(ByVal p As Integer)MessageBox.Show(String.Format("The parameter passed to command is {0}.", p))End SubPublic Function DoSomethingCriteria(ByVal p As Integer) As BooleanReturn (2 + 2) = pEnd Function
当命令刚刚绑定到它的目标(以获取目标的初始状态)时,首先检查 CanExecute 子句。 稍后,每次 CanExecuteChanged 事件通知命令的目标有关命令状态更改时,都会重新计算此条件。 此事件是在底层命令对象级别声明的,要从 ViewModel 级别发送此类通知,请按如下方式调用 RaiseCanExecuteChanged 扩展方法。
C#
//a bindable propertypublic virtual bool IsModified { get; protected set; }//a commandpublic void Save() {//. . .}//a CanExecute conditionpublic bool CanSave() {return IsModified;}//the OnChanged method calls the RaiseCanExecuteChanged method for the "Save" command//this forces the command to update its CanExecute conditionpublic void OnIsModifiedChanged() {this.RaiseCanExecuteChanged(x=>x.Save());}
VB.NET
'a bindable propertyPrivate privateIsModified As BooleanPublic Overridable Property IsModified() As BooleanGetReturn privateIsModifiedEnd GetProtected Set(ByVal value As Boolean)privateIsModified = valueEnd SetEnd Property'a commandPublic Sub Save()'. . .End Sub'a CanExecute conditionPublic Function CanSave() As BooleanReturn IsModifiedEnd Function'the OnChanged method calls the RaiseCanExecuteChanged method for the "Save" command'this forces the command to update its CanExecute conditionPublic Sub OnIsModifiedChanged()Me.RaiseCanExecuteChanged(Sub(x) x.Save())End Sub
服务
为了解析服务,框架会覆盖接口类型的虚拟属性,这些属性的名称必须以 …Service 结尾。
C#
public virtual IMyNotificationService MyService {get { throw new NotImplementedException(); }}public virtual IMyNotificationService AnotherService {get { throw new NotImplementedException(); }}
VB.NET
Public Overridable ReadOnly Property MyService() As IMyNotificationServiceGetThrow New NotImplementedException()End GetEnd PropertyPublic Overridable ReadOnly Property AnotherService() As IMyNotificationServiceGetThrow New NotImplementedException()End GetEnd Property
您还可以使用 ServiceProperty 属性显式标记具有其他名称的服务属性。
C#
using DevExpress.Mvvm.DataAnnotations;//. . .[ServiceProperty]public virtual IMyNotificationService MyProvider {get { throw new NotImplementedException(); }}
VB.NET
Imports DevExpress.Mvvm.DataAnnotations'. . .<ServiceProperty>Public Overridable ReadOnly Property MyProvider() As IMyNotificationServiceGetThrow New NotImplementedException()End GetEnd Property
当框架覆盖服务属性时,它会生成相应的 GetService<> 扩展方法调用。 ServiceProperty 属性允许您为此方法指定其他参数(例如,服务密钥)。
C#
[ServiceProperty(Key="Service1")]public virtual IMyNotificationService Service {get { throw new NotImplementedException(); }}[ServiceProperty(Key = "Service2")]public virtual IMyNotificationService AnotherService {get { throw new NotImplementedException(); }}
VB.NET
<ServiceProperty(Key:="Service1")>Public Overridable ReadOnly Property Service() As IMyNotificationServiceGetThrow New NotImplementedException()End GetEnd Property<ServiceProperty(Key := "Service2")>Public Overridable ReadOnly Property AnotherService() As IMyNotificationServiceGetThrow New NotImplementedException()End GetEnd Property
DevExpress WinForm | 下载试用
DevExpress WinForm拥有180+组件和UI库,能为Windows Forms平台创建具有影响力的业务解决方案。DevExpress WinForms能完美构建流畅、美观且易于使用的应用程序,无论是Office风格的界面,还是分析处理大批量的业务数据,它都能轻松胜任!
更多产品正版授权详情及优惠,欢迎咨询在线客服>>
DevExpress技术交流群4:715863792 欢迎一起进群讨论
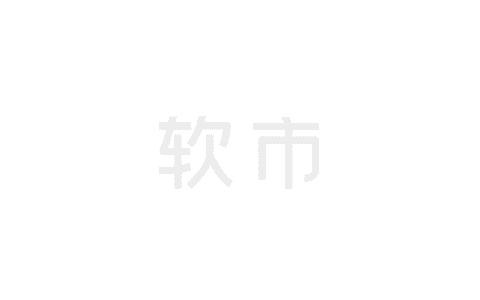
标签:
声明:本站部分文章及图片源自用户投稿,如本站任何资料有侵权请您尽早请联系jinwei@zod.com.cn进行处理,非常感谢!