(一) Java OMR API 从图像中提取数据
为了执行 OMR 操作并从支持的图像格式中提取数据,我们将使用Aspose.OMR for Java API。它允许设计、创建和识别答题纸、测试、MCQ 试卷、测验、反馈表、调查和选票。
API的OmrEngine类处理模板的创建和图像处理。此类的getTemplateProcessor(String templatePath)方法创建TemplateProcessor实例用于处理模板和图像。我们可以使用识别图像(字符串图像路径)方法识别图像。它将所有 OMR 元素作为RecognitionResult类实例返回。该类的getCsv()方法生成带有识别结果的CSV字符串。recalculate(RecognitionResult result, int recognitionThreshold)方法使用微调参数更新识别结果。
请下载 API 的 JAR或在基于 Maven 的 Java 应用程序中添加以下pom.xml配置。
<repository><id>AsposeJavaAPI</id><name>Aspose Java API</name><url>http://repository.aspose.com/repo/</url></repository><dependency>
<dependency><groupId>com.aspose</groupId><artifactId>aspose-omr</artifactId><version>19.12</version></dependency>
(二) 用Java从图像中提取数据
我们需要准备好的 OMR 模板 (.omr) 以及用户填写的表格/表格的图像来执行 OMR 操作。我们可以按照以下步骤对图像执行 OMR 操作并提取数据:
- 首先,创建OmrEngine类的实例。
- 接下来,调用getTemplateProcessor()方法并初始化TemplateProcessor类对象。它将 OMR 模板文件路径作为参数。
- 然后,通过以图像路径为参数调用recognitionImage()方法来获取RecognitionResult对象。
- 之后,使用getCsv()方法将识别结果作为 CSV 字符串获取。
- 最后,将 CSV 结果保存为本地磁盘上的 CSV 文件。
以下代码示例展示了如何使用 Java 从 CSV 格式的图像中提取 OMR 数据。
// This code example demonstrates how to perform OMR on an image and extract data// OMR Template file pathString templatePath = "C:FilesOMRSheet.omr";// Image file pathString imagePath = "C:FilesOMRSheet1.png";// Initialize OMR EngineOmrEngine engine = new OmrEngine();// Get template processorTemplateProcessor templateProcessor = engine.getTemplateProcessor(templatePath);// Recognize imageRecognitionResult result = templateProcessor.recognizeImage(imagePath);// Get results in CSVString csvResult = result.getCsv();// Save CSV filePrintWriter wr = new PrintWriter(new FileOutputStream("C:FilesOMRSheet1.csv"), true);wr.println(csvResult);
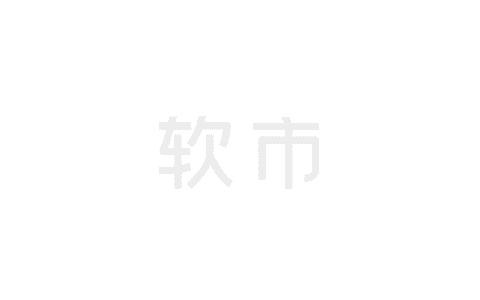
请下载本博文中使用的 OMR 模板。
(三) 执行 OMR 并从多个图像中提取数据
我们可以对多个图像执行 OMR 操作,并按照前面提到的步骤将数据提取到每个图像的单独 CSV 文件中。但是,我们需要对所有图像一张一张地重复步骤 #3、4 和 5。
以下代码示例展示了如何使用 Java 从多个图像中提取 OMR 数据。
// This code example demonstrates how to perform OMR on multiple images and extract data// Working folder pathString folderPath = "C:FilesOMR";// OMR Template file pathString templatePath = folderPath + "Sheet.omr";// Image file pathString[] UserImages = new String[] { "Sheet1.png", "Sheet2.png" } ;// Initialize OMR EngineOmrEngine engine = new OmrEngine();// Get template processorTemplateProcessor templateProcessor = engine.getTemplateProcessor(templatePath);// Process images one by one in a loopfor (int i = 0; i < UserImages.length; i++){String image = UserImages[i];String imagePath = folderPath + image;// Recognize imageRecognitionResult result = templateProcessor.recognizeImage(imagePath);// Get results in CSVString csvResult = result.getCsv();// Save CSV filePrintWriter wr = new PrintWriter(new FileOutputStream(folderPath + "Sheet_" + i + ".csv"), true);wr.println(csvResult);System.out.println(csvResult);}
(四) 在 Java 中提取具有阈值的 OMR 数据
我们可以根据要求执行具有阈值(0 到 100)的 OMR 操作。阈值越高,API 在突出显示答案时就越严格。请按照前面提到的步骤使用阈值执行 OMR。但是,我们只需要在步骤#3 中调用重载的recognizeImage(string, int32)方法。它将图像文件路径和阈值作为参数。
以下代码示例显示了如何使用 Java 执行具有阈值的 OMR。
// This code example demonstrates how to perform OMR with therashold and extract data from an image// OMR Template file pathString templatePath = "C:FilesOMRSheet.omr";// Image file pathString imagePath = "C:FilesOMRSheet1.png";// Threshold valueint CustomThreshold = 40;// Initialize OMR EngineOmrEngine engine = new OmrEngine();// Get template processorTemplateProcessor templateProcessor = engine.getTemplateProcessor(templatePath);// Recognize imageRecognitionResult result = templateProcessor.recognizeImage(imagePath, CustomThreshold);// Get results in CSVString csvResult = result.getCsv();// Save CSV filePrintWriter wr = new PrintWriter(new FileOutputStream("C:FilesOMRSheet1_threshold.csv"), true);wr.println(csvResult);System.out.println(csvResult);
(五)用 Java 重新计算提取 OMR 数据
在某些情况下,我们可能需要使用不同的阈值重新计算 OMR 结果。为此,我们可以将 API 配置为使用TemplateProcessor.recalculate()方法自动重新计算。它允许通过更改阈值设置来多次处理图像以获得所需的结果。我们可以按照以下给出的步骤执行带有重新计算的 OMR 操作:
- 首先,创建OmrEngine类的实例。
- 接下来,调用getTemplateProcessor()方法并初始化TemplateProcessor类对象。它将 OMR 模板文件路径作为参数。
- 然后,通过以图像路径为参数调用recognitionImage()方法来获取RecognitionResult对象。
- 接下来,使用getCsv()方法将识别结果导出为 CSV 字符串。
- 然后,将 CSV 结果保存为本地磁盘上的 CSV 文件。
- 接下来,调用recalculate()方法。它将 RecognitionResult 对象和阈值作为参数。
- 之后,使用getCsv()方法将识别结果导出为 CSV 字符串。
- 最后,将 CSV 结果保存为本地磁盘上的 CSV 文件。
以下代码示例显示了如何使用 Java 使用重新计算方法执行 OMR。
// OMR Template file pathString templatePath = "C:FilesOMRSheet.omr";// Image file pathString imagePath = "C:FilesOMRSheet1.png";// Threshold valueint CustomThreshold = 40;// Initialize OMR EngineOmrEngine engine = new OmrEngine();// Get template processorTemplateProcessor templateProcessor = engine.getTemplateProcessor(templatePath);// Recognize imageRecognitionResult result = templateProcessor.recognizeImage(imagePath, CustomThreshold);// Get results in CSVString csvResult = result.getCsv();// Save CSV filePrintWriter wr = new PrintWriter(new FileOutputStream("C:FilesOMRSheet1.csv"), true);wr.println(csvResult);// Recalculate// You may apply new threshold value heretemplateProcessor.recalculate(result, CustomThreshold);// Get recalculated results in CSVcsvResult = result.getCsv();// Save recalculated resultant CSV filePrintWriter finalWr = new PrintWriter(new FileOutputStream("C:FilesOMRSheet1_recalculated.csv"), true);finalWr.println(csvResult);
欢迎下载|体验更多Aspose产品
获取更多信息请咨询在线客服 或 加入Aspose技术交流群()
标签:
声明:本站部分文章及图片源自用户投稿,如本站任何资料有侵权请您尽早请联系jinwei@zod.com.cn进行处理,非常感谢!