带参数的命令
DevExpress MVVM框架接受public void方法的一个参数作为参数化命令,您可以使用此参数在 View 和 ViewModel 之间传递数据。
C#
//ViewModelpublic class ViewModelWithParametrizedCommand {public void DoSomething(object p) {var msgBoxService = this.GetService<IMessageBoxService>();msgBoxService.ShowMessage(string.Format("The parameter is {0}.", p));}}//ViewmvvmContext.ViewModelType = typeof(ViewModelWithParametrizedCommand);var fluent = mvvmContext.OfType<ViewModelWithParametrizedCommand>();object parameter = 5;fluent.BindCommand(commandButton, x => x.DoSomething, x => parameter);
VB.NET
'ViewModelPublic Class ViewModelWithParametrizedCommandPublic Sub DoSomething(ByVal p As Object)Dim msgBoxService = Me.GetService(Of IMessageBoxService)()msgBoxService.ShowMessage(String.Format("The parameter is {0}.", p))End SubEnd Class'ViewmvvmContext.ViewModelType = GetType(ViewModelWithParametrizedCommand)Dim fluent = mvvmContext.OfType(Of ViewModelWithParametrizedCommand)()Dim parameter As Object = 5fluent.BindCommand(commandButton, Sub(x) x.DoSomething(Nothing), Function(x) parameter)
您还可以向 CanExecute 条件添加参数。
C#
//ViewModelpublic class ViewModelWithParametrizedConditionalCommand {public void DoSomething(int p) {var msgBoxService = this.GetService<IMessageBoxService>();msgBoxService.ShowMessage(string.Format("The parameter is {0}.", p));}public bool CanDoSomething(int p) {return (2 + 2) == p;}}//ViewmvvmContext.ViewModelType = typeof(ViewModelWithParametrizedConditionalCommand);var fluent = mvvmContext.OfType<ViewModelWithParametrizedConditionalCommand>();int parameter = 4;fluent.BindCommand(commandButton, x => x.DoSomething, x => parameter);
VB.NET
'ViewModelPublic Class ViewModelWithParametrizedConditionalCommandPublic Sub DoSomething(ByVal p As Integer)Dim msgBoxService = Me.GetService(Of IMessageBoxService)()msgBoxService.ShowMessage(String.Format("The parameter is {0}.", p))End SubPublic Function CanDoSomething(ByVal p As Integer) As BooleanReturn (2 + 2) = pEnd FunctionEnd Class'ViewmvvmContext.ViewModelType = GetType(ViewModelWithParametrizedConditionalCommand)Dim fluent = mvvmContext.OfType(Of ViewModelWithParametrizedConditionalCommand)()Dim parameter As Integer = 4fluent.BindCommand(commandButton, Sub(x) x.DoSomething(Nothing), Function(x) parameter)
异步命令
如果需要执行延迟或连续操作,请使用异步命令。 要创建异步命令,请声明 System.Threading.Tasks.Task 类型的公共方法(您可以使用 async/await 语法),将 UI 元素绑定到命令的代码保持不变,框架在命令运行时禁用此元素。
C#
//ViewModelpublic class ViewModelWithAsyncCommand {public async Task DoSomethingAsync() {// do some work hereawait Task.Delay(1000);}}//ViewmvvmContext.ViewModelType = typeof(ViewModelWithAsyncCommand);var fluent = mvvmContext.OfType<ViewModelWithAsyncCommand>();fluent.BindCommand(commandButton, x => x.DoSomethingAsync);
VB.NET
'ViewModelPublic Class ViewModelWithAsyncCommandPublic Async Sub DoSomethingAsync() As Task' do some work hereAwait Task.Delay(1000)End SubEnd Class'ViewmvvmContext.ViewModelType = GetType(ViewModelWithAsyncCommand)Dim fluent = mvvmContext.OfType(Of ViewModelWithAsyncCommand)()fluent.BindCommand(commandButton, Sub(x) x.DoSomethingAsync(Nothing))
任务支持取消标记,允许您检查 IsCancellationRequested 属性并在此属性返回 true 时中止任务。 如果将此代码添加到异步命令中,请使用 BindCancelCommand 方法创建停止正在进行的异步命令的 UI 元素。 DevExpress MVVM 框架锁定了这个取消按钮,并且只有在相关的异步命令正在运行时才启用它。
C#
//ViewModelpublic class ViewModelWithAsyncCommandAndCancellation {public async Task DoSomethingAsynchronously() {var dispatcher = this.GetService<IDispatcherService>();var asyncCommand = this.GetAsyncCommand(x => x.DoSomethingAsynchronously());for(int i = 0; i <= 100; i++) {if(asyncCommand.IsCancellationRequested)break;// do some work hereawait Task.Delay(25);await UpdateProgressOnUIThread(dispatcher, i);}await UpdateProgressOnUIThread(dispatcher, 0);}public int Progress {get;private set;}//update the "Progress" property bound to the progress bar within a Viewasync Task UpdateProgressOnUIThread(IDispatcherService dispatcher, int progress) {await dispatcher.BeginInvoke(() => {Progress = progress;this.RaisePropertyChanged(x => x.Progress);});}}//ViewmvvmContext.ViewModelType = typeof(ViewModelWithAsyncCommandAndCancellation);var fluent = mvvmContext.OfType<ViewModelWithAsyncCommandAndCancellation>();fluent.BindCommand(commandButton, x => x.DoSomethingAsynchronously);fluent.BindCancelCommand(cancelButton, x => x.DoSomethingAsynchronously);fluent.SetBinding(progressBar, p => p.EditValue, x => x.Progress);
VB.NET
'ViewModelPublic Class ViewModelWithAsyncCommandAndCancellationPublic Async Sub DoSomethingAsynchronously() As TaskDim dispatcher = Me.GetService(Of IDispatcherService)()Dim asyncCommand = Me.GetAsyncCommand(Sub(x) x.DoSomethingAsynchronously())For i As Integer = 0 To 100If asyncCommand.IsCancellationRequested ThenExit ForEnd If' do some work hereAwait Task.Delay(25)Await UpdateProgressOnUIThread(dispatcher, i)Next iAwait UpdateProgressOnUIThread(dispatcher, 0)End SubPrivate privateProgress As IntegerPublic Property Progress() As IntegerGetReturn privateProgressEnd GetPrivate Set(ByVal value As Integer)privateProgress = valueEnd SetEnd Property'update the "Progress" property bound to the progress bar within a ViewPrivate Async Sub UpdateProgressOnUIThread(ByVal dispatcher As IDispatcherService, ByVal progress As Integer) As TaskAwait dispatcher.BeginInvoke(Sub()Me.Progress = progressMe.RaisePropertyChanged(Sub(x) x.Progress)End Sub)End SubEnd Class'ViewmvvmContext.ViewModelType = GetType(ViewModelWithAsyncCommandAndCancellation)Dim fluent = mvvmContext.OfType(Of ViewModelWithAsyncCommandAndCancellation)()fluent.BindCommand(commandButton, Sub(x) x.DoSomethingAsynchronously)fluent.BindCancelCommand(cancelButton, Sub(x) x.DoSomethingAsynchronously)fluent.SetBinding(progressBar, Sub(p) p.EditValue, Sub(x) x.Progress)
WithCommand Fluent API 方法还支持可取消的异步命令。
C#
mvvmContext.ViewModelType = typeof(ViewModelWithAsyncCommandAndCancellation);// Initialize the Fluent APIvar fluent = mvvmContext.OfType<ViewModelWithAsyncCommandAndCancellation>();// Binding for buttonsfluent.WithCommand(x => x.DoSomethingAsynchronously).Bind(commandButton).BindCancel(cancelButton);
VB.NET
mvvmContext.ViewModelType = GetType(ViewModelWithAsyncCommandAndCancellation)' Initialize the Fluent APIDim fluent = mvvmContext.OfType(Of ViewModelWithAsyncCommandAndCancellation)()' Binding for buttonsfluent.WithCommand(Sub(x) x.DoSomethingAsynchronously).Bind(commandButton).BindCancel(cancelButton)
DevExpress WinForm | 下载试用
DevExpress WinForm拥有180+组件和UI库,能为Windows Forms平台创建具有影响力的业务解决方案。DevExpress WinForms能完美构建流畅、美观且易于使用的应用程序,无论是Office风格的界面,还是分析处理大批量的业务数据,它都能轻松胜任!
更多产品正版授权详情及优惠,欢迎咨询在线客服>>
DevExpress技术交流群5:742234706 欢迎一起进群讨论
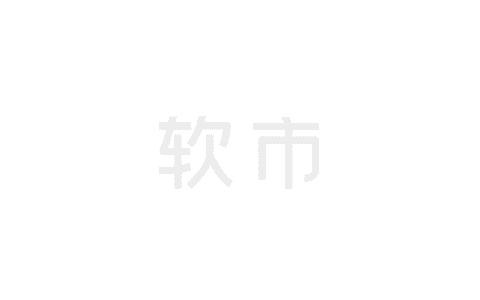
标签:
声明:本站部分文章及图片源自用户投稿,如本站任何资料有侵权请您尽早请联系jinwei@zod.com.cn进行处理,非常感谢!