>>你可以点击这里下载Aspose.Slides 最新版测试体验。
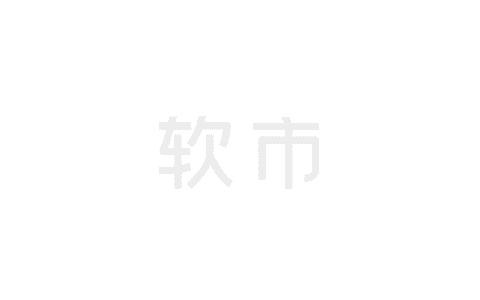
用于在 PowerPoint 演示文稿中创建和操作表格的 C++ API
我们将使用 Aspose.Slides for C++ API 来创建和操作 PowerPoint 演示文稿中的表格。它是一个强大且功能丰富的 API,支持创建、阅读和修改 PowerPoint 文件,而无需安装 Mircosoft PowerPoint。
使用 C++ 在 PowerPoint 演示文稿中创建表格
以下是在 PowerPoint 演示文稿中创建表格的步骤。
- 首先,创建一个Presentation 类的实例 来表示一个新的 PowerPoint 文件。
- 检索要添加表格的幻灯片。
- 在数组中,定义表格的宽度和高度。
- 使用ISlide->get_Shapes()->AddTable(float x, float y, System::ArrayPtr<double> columnWidths, System::ArrayPtr<double> rowHeights)方法创建表格。
- 创建一个循环来遍历表的行。
- 在循环内,创建一个嵌套循环以遍历每一行的单元格。
- 根据您的要求格式化单元格。
- 最后,使用 Presentation->Save(System::String fname, Export::SaveFormat format) 方法保存演示文稿。
以下示例代码显示了如何使用 C++ 在 PowerPoint 演示文稿中创建表格。
// File pathconst String outputFilePath = u"OutputDirectory\CreateTable_out.pptx";// Create an instance of the Presentation classauto presentation = System::MakeObject<Presentation>();// Access first slideSharedPtr<ISlide> slide = presentation->get_Slides()->idx_get(0);// Define columns with widths and rows with heightsSystem::ArrayPtr<double> dblCols = System::MakeObject<System::Array<double>>(4, 70);System::ArrayPtr<double> dblRows = System::MakeObject<System::Array<double>>(4, 70);// Add table shape to slideSharedPtr<ITable> table = slide->get_Shapes()->AddTable(100, 50, dblCols, dblRows);// Set border format for each cellfor (int x = 0; x < table->get_Rows()->get_Count(); x++){SharedPtr<IRow> row = table->get_Rows()->idx_get(x);for (int y = 0; y < row->get_Count(); y++){SharedPtr<ICell> cell = row->idx_get(y);cell->get_CellFormat()->get_BorderTop()->get_FillFormat()->set_FillType(FillType::Solid);cell->get_CellFormat()->get_BorderTop()->get_FillFormat()->get_SolidFillColor()->set_Color(System::Drawing::Color::get_Red());cell->get_CellFormat()->get_BorderTop()->set_Width(5);cell->get_CellFormat()->get_BorderBottom()->get_FillFormat()->set_FillType(FillType::Solid);cell->get_CellFormat()->get_BorderBottom()->get_FillFormat()->get_SolidFillColor()->set_Color(System::Drawing::Color::get_Red());cell->get_CellFormat()->get_BorderBottom()->set_Width(5);cell->get_CellFormat()->get_BorderLeft()->get_FillFormat()->set_FillType(FillType::Solid);cell->get_CellFormat()->get_BorderLeft()->get_FillFormat()->get_SolidFillColor()->set_Color(System::Drawing::Color::get_Red());cell->get_CellFormat()->get_BorderLeft()->set_Width(5);cell->get_CellFormat()->get_BorderRight()->get_FillFormat()->set_FillType(FillType::Solid);cell->get_CellFormat()->get_BorderRight()->get_FillFormat()->get_SolidFillColor()->set_Color(System::Drawing::Color::get_Red());cell->get_CellFormat()->get_BorderRight()->set_Width(5);}}// Save Presentationpresentation->Save(outputFilePath, Aspose::Slides::Export::SaveFormat::Pptx);
示例代码生成的输出图像:
使用 C++ 访问和修改 PowerPoint 演示文稿中的表格
您还可以访问和修改 PowerPoint 演示文稿中的现有表格。以下是访问和修改 PowerPoint 演示文稿中的表格的步骤。
- 首先,使用Presentation 类加载 PowerPoint 文件 。
- 检索包含表格的幻灯片。
- 遍历幻灯片的形状。
- 如果形状是ITable类型,则将其转换为ITable并将其存储在变量中。
- 使用ITable->idx_get(int32_t columnIndex, int32_t rowIndex)->get_TextFrame()->set_Text(System::String value)方法更新表的文本。
- 最后,使用 Presentation->Save(System::String fname, Export::SaveFormat format) 方法保存演示文稿。
以下示例代码显示了如何使用 C++ 访问和修改 PowerPoint 演示文稿中的表格。
// File pathsconst String sourceFilePath = u"OutputDirectory\CreateTable_out.pptx";const String outputFilePath = u"OutputDirectory\AccessTable_out.pptx";// Load the presentation fileauto presentation = System::MakeObject<Presentation>(sourceFilePath);// Access first slideSharedPtr<ISlide> slide = presentation->get_Slides()->idx_get(0);// Access the tableSharedPtr<ITable> table;for (SharedPtr<IShape> shape : slide->get_Shapes()){if (System::ObjectExt::Is<ITable>(shape)) {table = System::DynamicCast_noexcept<ITable>(shape);}}// Set texttable->idx_get(0, 1)->get_TextFrame()->set_Text(u"Aspose");// Save Presentationpresentation->Save(outputFilePath, Aspose::Slides::Export::SaveFormat::Pptx);
使用 C++ 在 PowerPoint 表格中设置文本方向
以下是在 PowerPoint 表格中设置文本方向的步骤。
- 首先,使用Presentation 类加载 PowerPoint 文件 。
- 检索包含表格的幻灯片。
- 遍历幻灯片的形状。
- 如果形状是ITable类型,则将其转换为ITable并将其存储在变量中。
- 使用ITable->idx_get(int32_t columnIndex, int32_t rowIndex)方法访问ICell对象中表格的所需单元格。
- 使用ICell->set_TextAnchorType(Aspose::Slides::TextAnchorType value)方法设置文本锚点类型。
- 使用ICell->set_TextVerticalType(Aspose::Slides::TextVerticalType value)方法设置文本方向。
- 最后,使用 Presentation->Save(System::String fname, Export::SaveFormat format) 方法保存演示文稿。
以下示例代码显示了如何使用 C++ 设置 PowerPoint 表格中文本的方向。
// File pathsconst String sourceFilePath = u"SourceDirectory\Slides\PresentationWithTable.pptx";const String outputFilePath = u"OutputDirectory\SetTextDirectionInTable_out.pptx";// Load the presentation fileauto presentation = System::MakeObject<Presentation>(sourceFilePath);// Access first slideSharedPtr<ISlide> slide = presentation->get_Slides()->idx_get(0);// Access the tableSharedPtr<ITable> table;for (SharedPtr<IShape> shape : slide->get_Shapes()){if (System::ObjectExt::Is<ITable>(shape)) {table = System::DynamicCast_noexcept<ITable>(shape);}}// Set text directionSharedPtr<ICell> cell = table->idx_get(0, 1);cell->set_TextAnchorType(TextAnchorType::Center);cell->set_TextVerticalType(TextVerticalType::Vertical270);// Save Presentationpresentation->Save(outputFilePath, Aspose::Slides::Export::SaveFormat::Pptx);
示例代码生成的输出图像:
如果你想试用Aspose的全部完整功能,可联系在线客服获取30天临时授权体验。
还想要更多吗可以点击阅读【Aspose最新资源在线文库】,查找需要的教程资源。如果您有任何疑问或需求,请随时加入Aspose技术交流群(),我们很高兴为您提供查询和咨询。
标签:
声明:本站部分文章及图片源自用户投稿,如本站任何资料有侵权请您尽早请联系jinwei@zod.com.cn进行处理,非常感谢!