文章应涵盖以下主题:
- WPF条码生成器的特点
- C# 条码生成器 API
- 创建 WPF 条码生成器的步骤
- 生成带有附加选项的条码
- 演示 WPF 条码生成器
- 下载源代码
WPF条码生成器的特点
我们的 WPF 条码生成器将具有以下功能。
- 生成以下类型的条码符 :
- 代码128
- 代码 11
- 代码 39
- 二维码
- 数据矩阵
- EAN13
- EAN8
- ITF14
- PDF417
- 将生成的条码图像保存为以下格式:
- PNG
- JPEG
- BMP
- 预览生成的条形码图像。
C# 条码生成器 API
我们将使用Aspose.BarCode for .NET API 来生成条形码图像并在 WPF 应用程序中预览它们。它是一个功能丰富的 API,可让您生成、扫描和读取各种条形码类型。此外,它允许操纵生成的条形码的外观,例如背景颜色、条形颜色、旋转角度、x 尺寸、图像质量、分辨率、标题、大小等等。
创建 WPF 条码生成器的步骤
我们可以按照以下步骤在 WPF 应用程序中生成和显示条形码图像:
- 首先,创建一个新项目并选择WPF Application项目模板。
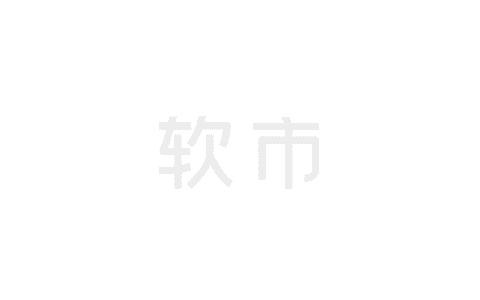
-
接下来,输入项目的名称,例如“BarcodeGen”。
-
然后,选择 .NET 框架,然后选择创建.
-
接下来,打开NuGet 包管理器并安装Aspose.BarCode for .NET包。
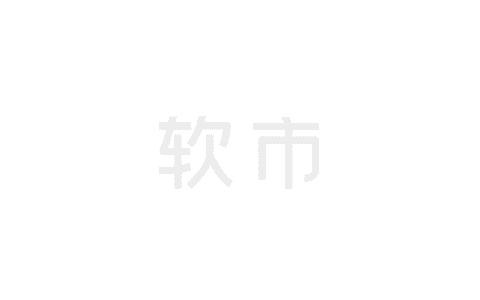
- 然后,添加一个新类Barcode.cs来定义条形码。
public class Barcode{public stringText { get; set; }public BaseEncodeTypeBarcodeType { get; set; }public BarCodeImageFormat ImageType { get; set; }}
- 接下来,打开MainWindow.xaml并添加所需的控件,如下所示:
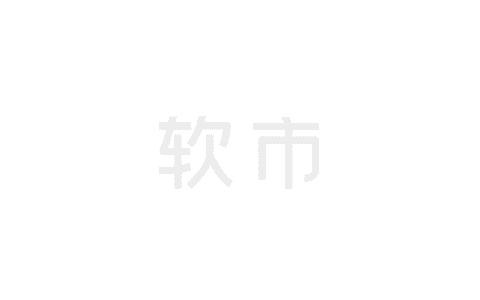
您还可以将MainWindow.xaml的内容替换为以下脚本。
<Window x_Class="BarcodeGen.MainWindow"xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"xmlns:d="http://schemas.microsoft.com/expression/blend/2008"xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"xmlns:local="clr-namespace:BarcodeGen"mc:Ignorable="d"Title="MainWindow" Height="450" Width="800"><Grid Width="800" Height="384"><Grid.RowDefinitions><RowDefinition Height="191*"/><RowDefinition/></Grid.RowDefinitions><Label Content="Select Barcode Type:" HorizontalAlignment="Left" Margin="10,16,0,0" VerticalAlignment="Top" FontSize="14" FontWeight="Bold"/><ComboBox x_Name="comboBarcodeType" HorizontalAlignment="Left" Margin="10,47,0,305" Width="273" Text="Select Barcode Type" IsReadOnly="True" SelectedIndex="-1" FontSize="14" Height="30"><ComboBoxItem Content="Code128"></ComboBoxItem><ComboBoxItem Content="Code11"></ComboBoxItem><ComboBoxItem Content="Code32"></ComboBoxItem><ComboBoxItem Content="QR"></ComboBoxItem><ComboBoxItem Content="DataMatrix"></ComboBoxItem><ComboBoxItem Content="EAN13"></ComboBoxItem><ComboBoxItem Content="EAN8"></ComboBoxItem><ComboBoxItem Content="ITF14"></ComboBoxItem><ComboBoxItem Content="PDF417"></ComboBoxItem></ComboBox><Button Name="btnGenerate" Click="btnGenerate_Click" Content="Generate Barcode" HorizontalAlignment="Left" Margin="10,346,0,0" VerticalAlignment="Top" Height="28" Width="273" FontSize="14" FontWeight="Bold"/><Label Content="Enter Your Text:" HorizontalAlignment="Left" Margin="10,92,0,0" VerticalAlignment="Top" FontSize="14" FontWeight="Bold"/><TextBox Name="tbCodeText" TextWrapping="Wrap" Margin="10,123,517,134" Width="273" Height="125"/><Label Content="Select Image Format:" HorizontalAlignment="Left" Margin="10,253,0,0" VerticalAlignment="Top" FontSize="14" FontWeight="Bold"/><RadioButton Name="rbPng" Content="Png" GroupName="rbImageType" Margin="10,285,739,77" Width="51" Height="20" FontSize="14" IsChecked="True"/><RadioButton Name="rbJpg" Content="Jpeg" GroupName="rbImageType" Margin="121,285,628,77" Width="51" Height="20" FontSize="14"/><RadioButton Name="rbBmp" Content="Bmp" GroupName="rbImageType" Margin="232,285,517,77" Width="51" Height="20" FontSize="14"/><CheckBox Name="cbGenerateWithOptions" Height="20" Margin="10,321,517,41" Content="Generate with Options" /><GroupBox Header="View Generated Barcode" Margin="317,0,22,0" FontSize="14" FontWeight="Bold"><Image Name="imgDynamic" Margin="6,-6,7,6" Stretch="None" /></GroupBox></Grid></Window>view rawWPF-Barcode-Generator_MainWindow.xaml hosted with by GitHub然后,打开MainWindow.xaml.cs类并添加btnGenerate_Click事件来处理Generate Barcode按钮的单击操作。private void btnGenerate_Click(object sender, RoutedEventArgs e){// Set default as Pngvar imageType = "Png";// Get the user selected image formatif(rbPng.IsChecked == true){imageType = rbPng.Content.ToString();}else if(rbBmp.IsChecked == true){imageType = rbBmp.Content.ToString();}else if(rbJpg.IsChecked == true){imageType = rbJpg.Content.ToString();}// Get image format from enumvar imageFormat = (BarCodeImageFormat)Enum.Parse(typeof(BarCodeImageFormat), imageType.ToString());// Set default as Code128var encodeType = EncodeTypes.Code128;// Get the user selected barcode typeif (!string.IsNullOrEmpty(comboBarcodeType.Text)){switch (comboBarcodeType.Text){case "Code128":encodeType = EncodeTypes.Code128;break;case "ITF14":encodeType = EncodeTypes.ITF14;break;case "EAN13":encodeType = EncodeTypes.EAN13;break;case "Datamatrix":encodeType = EncodeTypes.DataMatrix;break;case "Code32":encodeType = EncodeTypes.Code32;break;case "Code11":encodeType = EncodeTypes.Code11;break;case "PDF417":encodeType = EncodeTypes.Pdf417;break;case "EAN8":encodeType = EncodeTypes.EAN8;break;case "QR":encodeType = EncodeTypes.QR;break;}}// Initalize barcode objectBarcode barcode = new Barcode();barcode.Text = tbCodeText.Text;barcode.BarcodeType = encodeType;barcode.ImageType = imageFormat;try{string imagePath = "";if (cbGenerateWithOptions.IsChecked == true){// Generate barcode with additional options and get the image pathimagePath = GenerateBarcodeWithOptions(barcode);}else{// Generate barcode and get image pathimagePath = GenerateBarcode(barcode);}// Display the imageUri fileUri = new Uri(Path.GetFullPath(imagePath));imgDynamic.Source = new BitmapImage(fileUri);}catch (Exception ex){MessageBox.Show(ex.Message);}}
- 之后,添加一个生成条形码的函数。
private string GenerateBarcode(Barcode barcode){// Image pathstring imagePath = comboBarcodeType.Text + "." + barcode.ImageType;// Initilize barcode generatorBarcodeGenerator generator = new BarcodeGenerator(barcode.BarcodeType, barcode.Text);// Save the imagegenerator.Save(imagePath, barcode.ImageType);return imagePath;}
- 最后,运行应用程序。
用 Java 自定义瑞士二维码
我们还可以生成带有特定于条形码类型的附加选项的条形码。在 WPF 条码生成器中,我们添加了一个复选框来生成带有选项的条码。它将调用以下函数,为不同的条形码类型指定附加选项。
private string GenerateBarcodeWithOptions(Barcode barcode){// Image pathstring imagePath = comboBarcodeType.Text + "." + barcode.ImageType;// Initilize barcode generatorBarcodeGenerator generator = new BarcodeGenerator(barcode.BarcodeType, barcode.Text);if(barcode.BarcodeType == EncodeTypes.QR){generator.Parameters.Barcode.XDimension.Pixels = 4;//set Auto versiongenerator.Parameters.Barcode.QR.QrVersion = QRVersion.Auto;//Set Auto QR encode typegenerator.Parameters.Barcode.QR.QrEncodeType = QREncodeType.Auto;}else if(barcode.BarcodeType == EncodeTypes.Pdf417){generator.Parameters.Barcode.XDimension.Pixels = 2;generator.Parameters.Barcode.Pdf417.Columns = 3;}else if(barcode.BarcodeType == EncodeTypes.DataMatrix){//set DataMatrix ECC to 140generator.Parameters.Barcode.DataMatrix.DataMatrixEcc = DataMatrixEccType.Ecc200;}else if(barcode.BarcodeType == EncodeTypes.Code32){generator.Parameters.Barcode.XDimension.Millimeters = 1f;}else{generator.Parameters.Barcode.XDimension.Pixels = 2;//set BarHeight 40generator.Parameters.Barcode.BarHeight.Pixels = 40;}// Save the imagegenerator.Save(imagePath, barcode.ImageType);return imagePath;}
生成带有附加选项的条码
我们还可以生成带有特定于条形码类型的附加选项的条形码。在 WPF 条码生成器中,我们添加了一个复选框来生成带有选项的条码。它将调用以下函数,为不同的条形码类型指定附加选项。
private string GenerateBarcodeWithOptions(Barcode barcode){// Image pathstring imagePath = comboBarcodeType.Text + "." + barcode.ImageType;// Initilize barcode generatorBarcodeGenerator generator = new BarcodeGenerator(barcode.BarcodeType, barcode.Text);if(barcode.BarcodeType == EncodeTypes.QR){generator.Parameters.Barcode.XDimension.Pixels = 4;//set Auto versiongenerator.Parameters.Barcode.QR.QrVersion = QRVersion.Auto;//Set Auto QR encode typegenerator.Parameters.Barcode.QR.QrEncodeType = QREncodeType.Auto;}else if(barcode.BarcodeType == EncodeTypes.Pdf417){generator.Parameters.Barcode.XDimension.Pixels = 2;generator.Parameters.Barcode.Pdf417.Columns = 3;}else if(barcode.BarcodeType == EncodeTypes.DataMatrix){//set DataMatrix ECC to 140generator.Parameters.Barcode.DataMatrix.DataMatrixEcc = DataMatrixEccType.Ecc200;}else if(barcode.BarcodeType == EncodeTypes.Code32){generator.Parameters.Barcode.XDimension.Millimeters = 1f;}else{generator.Parameters.Barcode.XDimension.Pixels = 2;//set BarHeight 40generator.Parameters.Barcode.BarHeight.Pixels = 40;}// Save the imagegenerator.Save(imagePath, barcode.ImageType);return imagePath;}
演示 WPF 条码生成器
下面是我们刚刚创建的 WPF Barcode Generator 应用程序的演示。
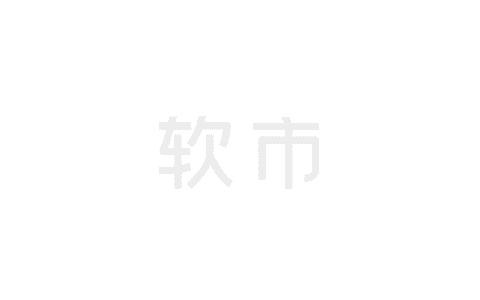
欢迎下载|体验更多Aspose产品
获取更多信息请咨询在线客服 或 加入Aspose技术交流群()
标签:
声明:本站部分文章及图片源自用户投稿,如本站任何资料有侵权请您尽早请联系jinwei@zod.com.cn进行处理,非常感谢!