在本教程中,我们将学习如何使用Firestore和官方的Highcharts Angular包装器可视化实时数据库更新。整个项目在此GitHub链接中。
Highcharts是一款纯JavaScript编写的图表库,为你的Web 站、Web应用程序提供直观、交互式图表。当前支持折线、曲线、区域、区域曲线图、柱形图、条形图、饼图、散点图、角度测量图、区域排列图、区域曲线排列图、柱形排列图、极坐标图等几十种图表类型。
Highcharts最新试用版
在本教程中,我们将学习如何使用Firestore和官方的Highcharts Angular包装器可视化实时数据库更新。整个项目在此GitHub链接中。
- 设置Cloud Firestore项目。
- 使用Firestore设置Angular项目。
- 将Highcharts与Angular和Firestore集成。
设置Cloud Firestore项目
1.要做的第一件事是创建一个项目,然后执行以下步骤:登录到https://console.firebase.google.com
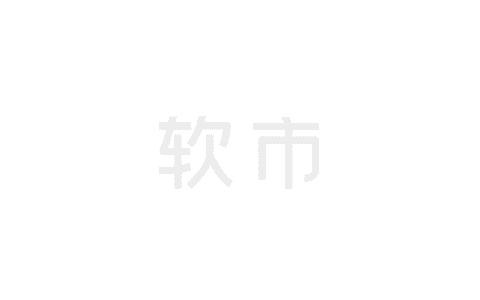
2.单击添加项目。
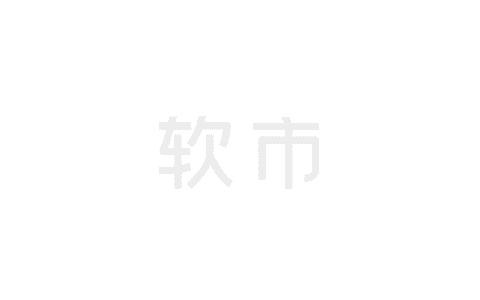
3.创建一个数据库。
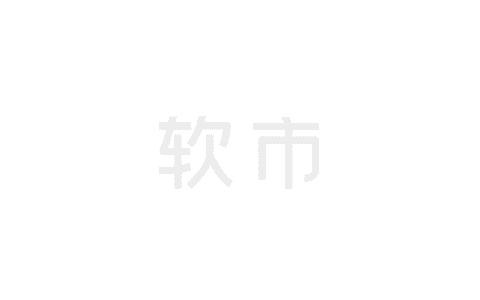
4.选择“以测试模式启动”。
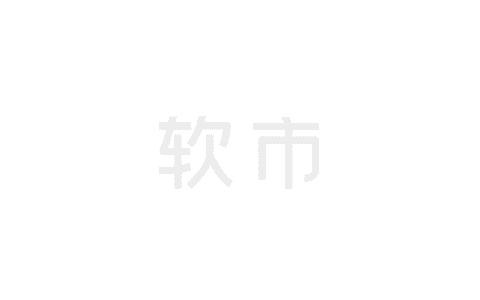
5.为数据库创建一个集合,使其具有多个文档来存储数据。
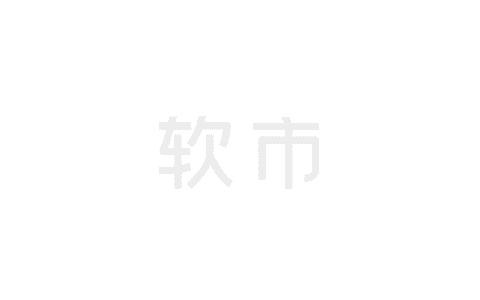
6.将文档添加到集合中。
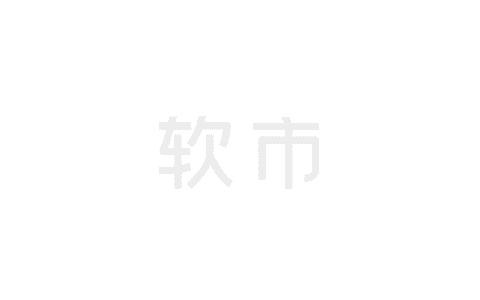
7.将您的Angular应用名称添加到Firestore中。请确保选择“ Web”,因为我们目前正在使用Web 。
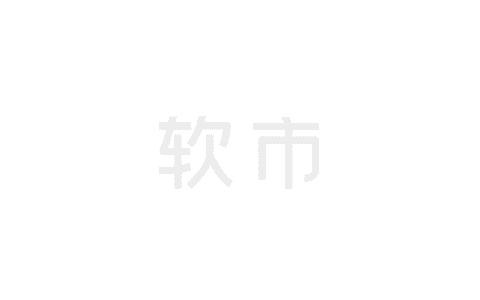
8.通过将Angular App Name注册到Firestore,我们将能够立即使用所有关键功能。
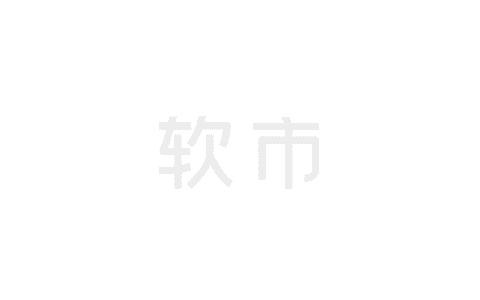
9.获取配置详细信息,然后firebaseConfig在angular应用程序中使用该对象。
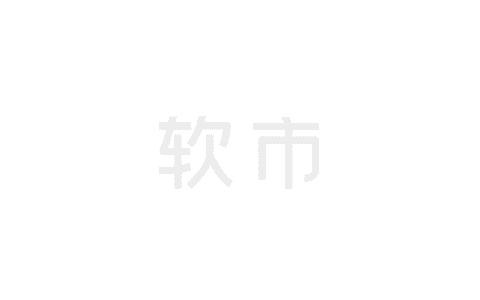
使用Firestore设置Angular项目
要设置Angular项目,请按照此链接中的说明进行操作。
顺便说一句,创建完Angular项目后,请不要忘记运行以下命令,并说明执行该操作的原因:ng add @angular/fire
- 列出所有Firebase项目。由此,我们可以选择我们的项目进行进一步的配置和开发。
- 创建一个列出您的项目配置的Firebase.json配置文件。
- 创建一个.firebaserc文件来存储您的项目别名。
- 要更新文件。angular.json
设置完成后,就该将Firebase配置对象添加到环境中了(见下文):
export const environment = { production: false, firebaseConfig: { apiKey: "AIzaSyBaGUX7iwBRthIKOE_uhyzs1aPEuKPlEAE", authDomain: "firestore-angular-highcharts.firebaseapp.com", databaseURL: "https://firestore-angular-highcharts.firebaseio.com", projectId: "firestore-angular-highcharts", storageBucket: "firestore-angular-highcharts.appspot.com", messagingSenderId: "669023523724", appId: "1:669023523724:web:43f04e6a2ce0a92c01fca9", measurementId: "G-ZD6C3CEC85" }};
在此HighchartService中与Cloud Firestore API进行交互的最后一步(请参见下文):
import { Injectable} from '@angular/core';import { Observable} from 'rxjs';import { AngularFirestore, AngularFirestoreCollection} from "@angular/fire/firestore";import { map} from "rxjs/operators";@Injectable({ providedIn: 'root'})export class HighchartService { private rateCollection: AngularFirestoreCollection < chartModal > ; rates$: Observable < chartModal[] > ; constructor(private readonly firestoreservice: AngularFirestore) { this.rateCollection = firestoreservice.collection < chartModal > ('ChartData'); // .snapshotChanges() returns a DocumentChangeAction[], which contains // a lot of information about "what happened" with each change. If you want to // get the data and the id use the map operator. this.rates$ = this.rateCollection.snapshotChanges().pipe( map(actions => actions.map(a => { const data = a.payload.doc.data() as chartModal; const id = a.payload.doc.id; return { id, ...data }; })) ); }}export interface chartModal { currency: string, rate: number}
将Highcharts与Angular和Firestore集成
为了使用Highcharts可视化我们项目上的数据更新,我们需要包含Highcharts Angular包装器。它允许我们访问highcharts库。是官方的角度包装器。要安装highcharts-angular和Highcharts库,只需运行以下指令:。要使用这些软件包,首先,必须导入它们。因此,在app.module.ts文件中,我们从highcharts-angular包中导入了模块。另外,我们导入并使用firebaseConfig(见下文):highchats-angular
npm install highcharts-angular highcharts
HighchartsChartModule
AngularFirestoreModuleinitializeApp
import { BrowserModule } from "@angular/platform-browser"; import { NgModule } from "@angular/core"; import { HighchartsChartModule } from "highcharts-angular"; import { AppRoutingModule } from "./app-routing.module"; import { AppComponent } from "./app.component"; import { AngularFireModule } from "@angular/fire"; import { environment } from "src/environments/environment"; import { AngularFireAnalyticsModule } from "@angular/fire/analytics"; import { AngularFirestoreModule } from "@angular/fire/firestore"; @NgModule({ declarations: [AppComponent], imports: [ BrowserModule, AppRoutingModule, HighchartsChartModule, AngularFireAnalyticsModule, AngularFirestoreModule, AngularFireModule.initializeApp(environment.firebaseConfig), ], providers: [], bootstrap: [AppComponent], }) export class AppModule {}
让我们来看一下app.component.ts中的操作。
Highcharts从导入。从导入服务和模式。上,我们从文件存储数据库订阅的发光值,并推动新值数组,然后调用和初始化图表数据。import * as Highcharts from “highcharts”;
highchart.service
ngOnInit()chartdatagetChart()
import { Component, OnInit} from "@angular/core";import { HighchartService, chartModal} from "./highchart.service";import * as Highcharts from "highcharts";@Component({ selector: "app-root", templateUrl: "./app.component.html", styleUrls: ["./app.component.css"],})export class AppComponent implements OnInit { title = "Firestore-Angular-Highcharts"; items$: chartModal[]; Highcharts: typeof Highcharts = Highcharts; chardata: any[] = []; chartOptions: any; constructor(private highchartservice: HighchartService) {} ngOnInit() { this.highchartservice.rates$.subscribe((assets) => { this.items$ = assets; if (this.items$) { this.items$.forEach((element) => { this.chardata.push(element.rate); }); this.getChart(); } }); } getChart() { this.chartOptions = { series: [{ data: this.chardata, }, ], chart: { type: "bar", }, title: { text: "barchart", }, }; }}
该项目的最后一步是转到app.component.html添加指令和一些属性绑定以初始化图表数据和选项:highcharts-chart
[Highcharts]=”Highcharts”
[options]=”chartOptions”
style=”width: 100%; height: 400px; display: block;”>
</highcharts-chart>
这是最终结果:
如下面的GIF所示,条形图从Firebase上托管的数据库中获取数据。当使用Firebase UI将新值添加到数据库时,图表的数据将更新并显示。
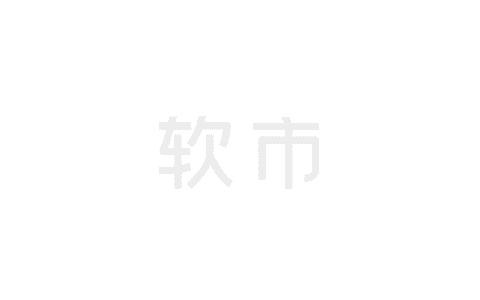
同样,在下面的示例中,我们正在将具有新值的折线图绘制到同一折线图上。
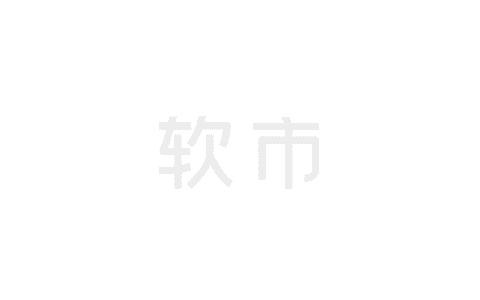
想要购买Highcharts正版授权,或了解更多产品信息请点击【咨询在线客服】
标签:
声明:本站部分文章及图片源自用户投稿,如本站任何资料有侵权请您尽早请联系jinwei@zod.com.cn进行处理,非常感谢!