一、实验环境
使用程序:putty.exe;
IntelliJ IDEA 2021.1.1;
apache-tomcat-9.0.46
服务器名称:ecs-d8b3
弹性公 :121.36.79.196
端口 :26000
表空间名:human_resource_space
数据库名称:human_resource
员工、部门经理登录账 :其员工ID
员工、部门经理登录密码:123456
人事经理登录账 :hr001
人事经理登录密码:hr001
登录入口(需在tomcat启动之后才能运行):http://localhost:8080/gaussdb2_war/login.jsp
二、创建和管理openGauss数据库
进行以下步骤前,需预先购买弹性云服务器 ECS ,并把需要的软件以及需要调用的包预先下载好。
2.1 数据库存储管理
2.1.1 连接弹性云服务器
我们使用 SSH 工具PuTTY,从本地电脑通过配置弹性云服务器的弹性公 IP地址来连接 ECS,并使用 ROOT 用户来登录。
(1)点击putty.exe,打开putty
2.1.2 启动、停止和连接数据库
2.1.1.1 启动数据库
(1)使用root登录
(3)启动数据库
使用语句启动数据库
gs_om -t start
图中使用 gsql -d postgres -p 26000 -r 连接postgres数据库。postgres 为 openGauss 安装完成后默认生成的数据库,初始可以连接到此数据库进行新数据库的创建。26000 为数据库主节点的端口 。
2.1.3 创建和管理用户、表空间、数据库和模式
2.1.3.1 创建用户
使用以下语句创建用户。请牢记设置的用户名以及密码,之后需要多次使用。建议将密码都设置为相同的简单密码,方便之后的操作。
CREATE USER user_name PASSWORD pass_word
2.1.3.2 管理用户
可以使用以下语句对用户进行操作:
修改密码:
ALTER USER a IDENTIFIED BY ‘Abcd@123′ REPLACE ‘Guass@123’;
删除用户:
DROP USER a CASCADE;
2.1.3.3 创建表空间
使用以下语句创建表空间。(路径需使用单引 )
CREATE TABLESPACE human_resource_space RELATIVE LOCATION ‘tablespace/tablespace_2’;
创建表空间 human_resource_space,表空间路径为:tablespace/tablespace_2
(2)管理表空间
如有需要,可以使用如下语句 或 db 语句查询表空间。
SELECT spcname FROM pg_tablespace;
可使用以下语句删除表空间
DROP TABLESPACE tablespace_1;
2.1.3.5 创建数据库
为用户a在表空间human_resource_space上创建数据库human_resource
CREATE DATABASE human_resource WITH TABLESPACE = human_resource_space OWNER a;
2.1.3.6 管理数据库
可以使用以下语句管理数据库:
SELECT datname FROM pg_database;
或 l 查看数据库
DROP DATABASE testdb;
删除数据库
2.1.3.7 创建模式
输入 q 退出postgres数据库。
使用语句
CREATE SCHEMA a AUTHORIZATION a;
为用户创建同名模式a
2.1.3.8 管理模式
SET SEARCH_PATH TO a,public;
设置模式a为默认查询模式(设置中第一个为默认模式)
如有需要,可以使用语句 dn 查看模式 ,SHOW SEARCH_PATH; 查看模式搜索路径
2.2 数据库对象管理实验
2.2.1 创建表
使用以下语句,在数据库human_resource_space,创建人力资源库的8个基本表。
CREATE TABLE table_name
( col1 datatype constraint,
col2 datatype constraint,
…
coln datatype constraint );
我们为了完成人力资源管理系统,创建雇佣历史表 employment_history 、部门表 sections、创建工作地点表 places、创建区域表 areas 、大学表 college、雇佣表 employments 、国家及地区表 states 、员工表 staffs这8个基本表。
以员工表为例:
3.1.1 BLL
业务逻辑层,实现各项操作模块与servlet的接口,对传送数据进行逻辑判断分折,并进行传送正确的值。
3.1.3 cn.UI
实现用户界面
3.1.4 Util
实现获得参数并传递参数以及连接数据库的功能
登录页面:
manager页面:
6.2 修改表staffs
为了实现登录功能,我们需要在员工表staffs中增加一列password,为了方便起见,我们设置密码都为123456,当然也可以自己设置差异化的密码。
ALTER TABLE staffs ADD password varchar2(20);
设置hr登录账 为hr001,密码为hr001
6.3 加载驱动&连接数据库
JDBC为JAVA中用来访问数据库的程序接口,我们使用JDBC连接。
文件路径为:
6.4 实现具体功能
文件路径:
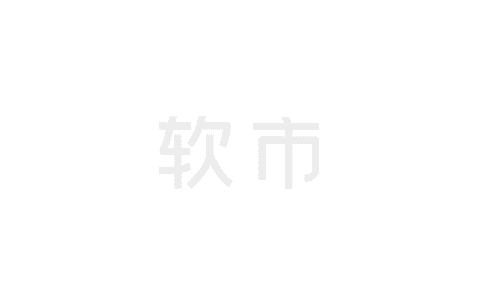
完整源码:
package Dao;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import Model.*;
import Util.getInformation;
public class operate {
//********************************登录**************************************
//实现登录操作,登录成功返回true
public String login(String staff_id,String password){
if(staff_id.equals("hr001")){
if (password.equals("hr001")){
return staff_id;
}else {
return null;
}
}else {
String sql="select staff_id,password from staffs ";
ResultSet rs=Util.getInformation.executeQuery(sql);
try {
while(rs.next()){ //用户输入的账 密码和数据库中的信息做比较,判断输入是否正确;
Integer id = rs.getInt("staff_id");
String pwd = rs.getString("password");
if(id.equals(new Integer(staff_id)) && pwd.equals(password)){
return staff_id;
}
}
rs.close();
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
return null;
}
//判断该员工是否为部门经理,返回部门编
public String isManager(String staff_id){
String sql="select section_id,manager_id from sections";
ResultSet rs=Util.getInformation.executeQuery(sql);
try {
while(rs.next()){ //用户输入的账 密码和数据库中的信息做比较,判断输入是否正确;
Integer id = rs.getInt("manager_id");
String section_id = rs.getString("section_id");
if(id.equals(new Integer(staff_id))){
return section_id;
}
}
rs.close();
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "null";
}
//**********************************员工操作***********************************
//修改电话 码
public void updatePhoneNumber(String phone_number,String staff_id){
String sql = "update staffs set phone_number=where staff_id=";
Util.getInformation.executeUpdate(sql, phone_number, new Integer(staff_id));
}
//**********************************部门经理**********************************
//查询部门所有员工信息(按员工编 升序排列)
public List QuerySectionStaffsOrderByStaffId(Integer section_id)
{
List list=new ArrayList(); //最终返回整个list集合
String sql="select * from staffs where section_id=order by staff_id asc";
ResultSet rs=getInformation.executeQuery(sql,section_id);
try {
while(rs.next())
{
//保存取出来的每一条记录
staffs staff =new staffs();
staff.setStaff_id(rs.getInt("staff_id"));
staff.setFirst_name(rs.getString("first_name"));
staff.setLast_name(rs.getString("last_name"));
staff.setEmail(rs.getString("email"));
staff.setPhone_number(rs.getString("phone_number"));
staff.setHire_date(rs.getDate("hire_date"));
staff.setEmployment_id(rs.getString("employment_id"));
staff.setSalary(rs.getInt("salary"));
staff.setCommission_pct(rs.getInt("commission_pct"));
staff.setManager_id(rs.getInt("manager_id"));
staff.setSection_id(rs.getInt("section_id"));
staff.setGraduated_name(rs.getString("graduated_name"));
staff.setPassword(rs.getString("password"));
list.add(staff);
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return list;
}
//查询部门所有员工信息(按工资降序排列)
public List QuerySectionStaffsOrderBySalary(Integer section_id)
{
List list=new ArrayList(); //最终返回整个list集合
String sql="select * from staffs where section_id=order by salary desc";
ResultSet rs=getInformation.executeQuery(sql,section_id);
try {
while(rs.next())
{
//保存取出来的每一条记录
staffs staff =new staffs();
staff.setStaff_id(rs.getInt("staff_id"));
staff.setFirst_name(rs.getString("first_name"));
staff.setLast_name(rs.getString("last_name"));
staff.setEmail(rs.getString("email"));
staff.setPhone_number(rs.getString("phone_number"));
staff.setHire_date(rs.getDate("hire_date"));
staff.setEmployment_id(rs.getString("employment_id"));
staff.setSalary(rs.getInt("salary"));
staff.setCommission_pct(rs.getInt("commission_pct"));
staff.setManager_id(rs.getInt("manager_id"));
staff.setSection_id(rs.getInt("section_id"));
staff.setGraduated_name(rs.getString("graduated_name"));
staff.setPassword(rs.getString("password"));
list.add(staff);
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return list;
}
//根据员工 查询部门内员工,然后返回该员工信息
public staffs QuerySectionStaffByStaff_id(Integer staff_id,Integer section_id)
{
staffs staff =new staffs();
String sql="select * from staffs where staff_id=and section_id=;
ResultSet rs=getInformation.executeQuery(sql, staff_id,section_id);
try {
if(rs.next())
{
staff.setStaff_id(rs.getInt("staff_id"));
staff.setFirst_name(rs.getString("first_name"));
staff.setLast_name(rs.getString("last_name"));
staff.setEmail(rs.getString("email"));
staff.setPhone_number(rs.getString("phone_number"));
staff.setHire_date(rs.getDate("hire_date"));
staff.setEmployment_id(rs.getString("employment_id"));
staff.setSalary(rs.getInt("salary"));
staff.setCommission_pct(rs.getInt("commission_pct"));
staff.setManager_id(rs.getInt("manager_id"));
staff.setSection_id(rs.getInt("section_id"));
staff.setGraduated_name(rs.getString("graduated_name"));
staff.setPassword(rs.getString("password"));
}
} catch (NumberFormatException | SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return staff;
}
//根据员工姓名查询部门内员工,然后返回该员工信息
public staffs QuerySectionStaffByFirstName(String first_name,Integer section_id)
{
staffs staff =new staffs();
String sql="select * from staffs where first_name=and section_id=;
ResultSet rs=getInformation.executeQuery(sql, first_name,section_id);
try {
if(rs.next())
{
staff.setStaff_id(rs.getInt("staff_id"));
staff.setFirst_name(rs.getString("first_name"));
staff.setLast_name(rs.getString("last_name"));
staff.setEmail(rs.getString("email"));
staff.setPhone_number(rs.getString("phone_number"));
staff.setHire_date(rs.getDate("hire_date"));
staff.setEmployment_id(rs.getString("employment_id"));
staff.setSalary(rs.getInt("salary"));
staff.setCommission_pct(rs.getInt("commission_pct"));
staff.setManager_id(rs.getInt("manager_id"));
staff.setSection_id(rs.getInt("section_id"));
staff.setGraduated_name(rs.getString("graduated_name"));
staff.setPassword(rs.getString("password"));
}
} catch (NumberFormatException | SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return staff;
}
public List SectionStatistics(String section_id)
{
ArrayList list =new ArrayList(); // 初始化
String sql="select avg(salary),min(salary),max(salary) from staffs where section_id = ";
ResultSet rs=getInformation.executeQuery(sql,section_id);
try {
while(rs.next())
{
//保存取出来的每一条记录
list.add(rs.getInt("avg"));
list.add(rs.getInt("max"));
list.add(rs.getInt("min"));
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return list;
}
//******************************人事经理操作*****************************************
//根据员工 查询员工,然后返回该员工信息
public staffs QueryStaffByStaff_id(Integer staff_id)
{
staffs staff =new staffs();
String sql="select * from staffs where staff_id=;
ResultSet rs=getInformation.executeQuery(sql, staff_id);
try {
if(rs.next())
{
staff.setStaff_id(rs.getInt("staff_id"));
staff.setFirst_name(rs.getString("first_name"));
staff.setLast_name(rs.getString("last_name"));
staff.setEmail(rs.getString("email"));
staff.setPhone_number(rs.getString("phone_number"));
staff.setHire_date(rs.getDate("hire_date"));
staff.setEmployment_id(rs.getString("employment_id"));
staff.setSalary(rs.getInt("salary"));
staff.setCommission_pct(rs.getInt("commission_pct"));
staff.setManager_id(rs.getInt("manager_id"));
staff.setSection_id(rs.getInt("section_id"));
staff.setGraduated_name(rs.getString("graduated_name"));
staff.setPassword(rs.getString("password"));
}
} catch (NumberFormatException | SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return staff;
}
//根据员工姓名查询员工,然后返回该员工信息
public staffs QueryStaffByFirstName(String first_name)
{
staffs staff =new staffs();
String sql="select * from staffs where first_name=;
ResultSet rs=getInformation.executeQuery(sql, first_name);
try {
if(rs.next())
{
staff.setStaff_id(rs.getInt("staff_id"));
staff.setFirst_name(rs.getString("first_name"));
staff.setLast_name(rs.getString("last_name"));
staff.setEmail(rs.getString("email"));
staff.setPhone_number(rs.getString("phone_number"));
staff.setHire_date(rs.getDate("hire_date"));
staff.setEmployment_id(rs.getString("employment_id"));
staff.setSalary(rs.getInt("salary"));
staff.setCommission_pct(rs.getInt("commission_pct"));
staff.setManager_id(rs.getInt("manager_id"));
staff.setSection_id(rs.getInt("section_id"));
staff.setGraduated_name(rs.getString("graduated_name"));
staff.setPassword(rs.getString("password"));
}
} catch (NumberFormatException | SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return staff;
}
//查询所有员工信息(按员工编 升序排列)
public List QueryAllStaffsOrderByStaffId()
{
List list=new ArrayList(); //最终返回整个list集合
String sql="select * from staffs order by staff_id asc";
ResultSet rs=getInformation.executeQuery(sql);
try {
while(rs.next())
{
//保存取出来的每一条记录
staffs staff =new staffs();
staff.setStaff_id(rs.getInt("staff_id"));
staff.setFirst_name(rs.getString("first_name"));
staff.setLast_name(rs.getString("last_name"));
staff.setEmail(rs.getString("email"));
staff.setPhone_number(rs.getString("phone_number"));
staff.setHire_date(rs.getDate("hire_date"));
staff.setEmployment_id(rs.getString("employment_id"));
staff.setSalary(rs.getInt("salary"));
staff.setCommission_pct(rs.getInt("commission_pct"));
staff.setManager_id(rs.getInt("manager_id"));
staff.setSection_id(rs.getInt("section_id"));
staff.setGraduated_name(rs.getString("graduated_name"));
staff.setPassword(rs.getString("password"));
list.add(staff);
声明:本站部分文章及图片源自用户投稿,如本站任何资料有侵权请您尽早请联系jinwei@zod.com.cn进行处理,非常感谢!